In case you have the dip angle and the azimuth angle (i.e. strike angle) of a plane, here is how to calculate the XYZ coordinates of 3 points of this plane.
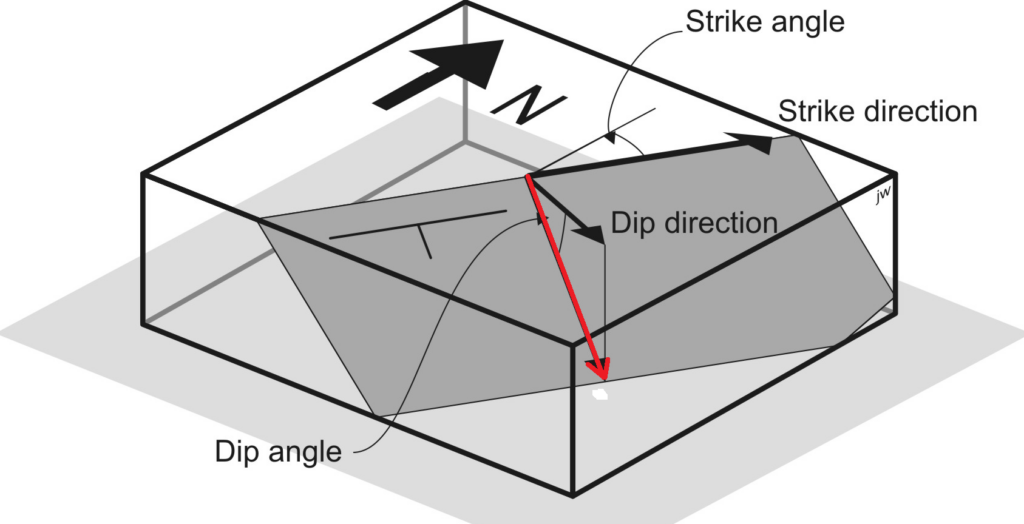
Note that below we assume that dip value is measured from horizontal plane. If dip is towards north, it must be set positive, and if it is towards south, set to negative.
Also, azimuth is measured positively in clockwise direction starting from North, i.e East is 90°, South is 180°…
In order to calculate coordinates of points belonging to this plane, let’s start from a point O (X0, Y0, Z0) as the origin.
Considering a distance d, a dip angle dip, and an azimuth (i.e. strike) angle az, we can use the spherical coordinates calculations to obtain the coordinates of a point A :
dXA = d*cos(az)*cos(dip)
dYA=d*sin(az)*cos(dip)
dZA=d*sin(dip)
XA=X0+dXA
YA=Y0+dYA
ZA=Z0+dZA
All angles must be set into radians.
And for a second point B on the horizontal direction (strike direction), we can do :
dXB=d*sin(az)
dYB=d*cos(az)
dZB=0
XB=X0+dXB
YB=Y0+dYB
ZB=Z0
Download a Calc file to do this here :
Usefull links to read also:
https://beckassets.blob.core.windows.net/product/readingsample/176217/9783540310549_excerpt_001.pdf
https://en.wikipedia.org/wiki/Spherical_coordinate_system#Cartesian_coordinates
https://gis.stackexchange.com/questions/13484/how-to-convert-distance-azimuth-dip-to-xyz